1.0 LLM API設計と実装ガイド | Flask & FastAPIチュートリアル
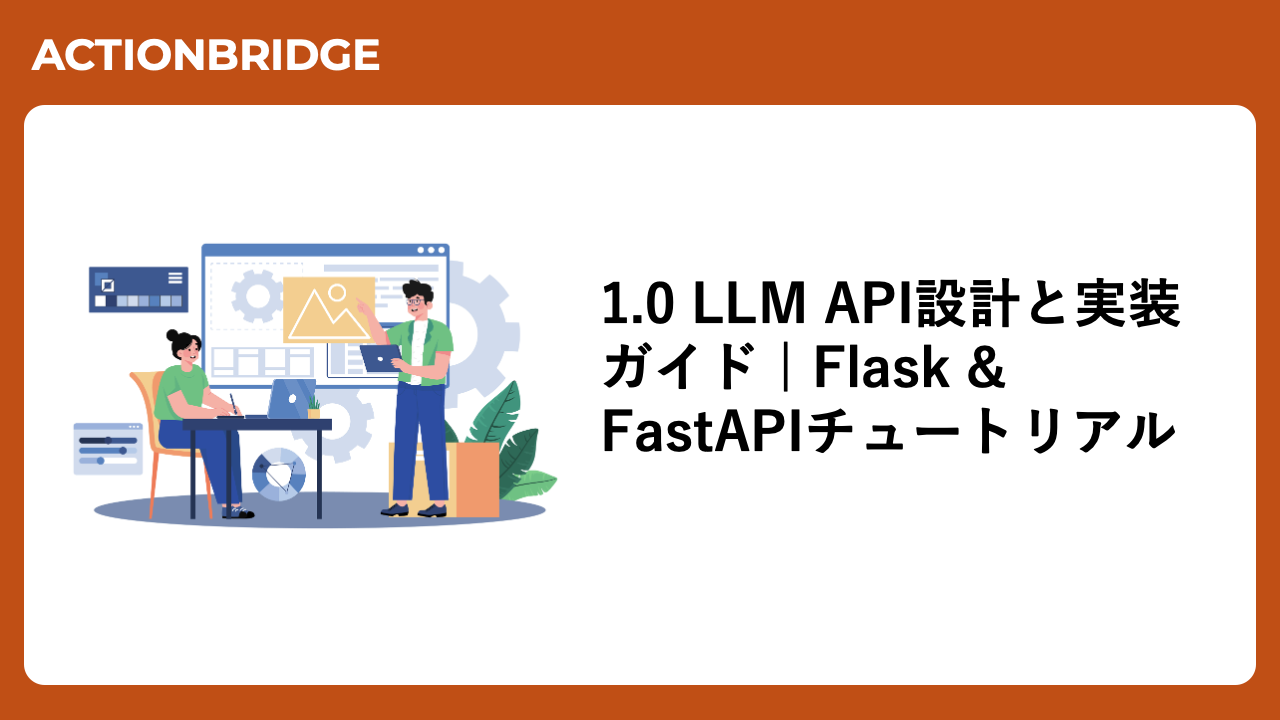
1.0 LLMを使ったAPIの設計と実装
このセクションでは、LLM(大規模言語モデル)を利用したAPIの設計と実装について解説します。FlaskやFastAPIなどのPythonフレームワークを活用し、効率的なAPIを構築する方法を学びます。また、LLM推論APIのスケーリングやキャッシュ戦略についても説明します。
1.1 FlaskまたはFastAPIを使ったAPIの基本設計
FlaskやFastAPIを使い、APIエンドポイントにLLM推論を組み込む方法を紹介します。
# FastAPIを使用したシンプルなLLM API例
from fastapi import FastAPI
from transformers import AutoModelForCausalLM, AutoTokenizer
app = FastAPI()
tokenizer = AutoTokenizer.from_pretrained("gpt2")
model = AutoModelForCausalLM.from_pretrained("gpt2")
@app.post("/predict")
async def predict(input_text: str):
input_ids = tokenizer.encode(input_text, return_tensors="pt")
output = model.generate(input_ids, max_length=50)
response_text = tokenizer.decode(output[0], skip_special_tokens=True)
return {"response": response_text}
1.2 LLM推論APIのスケーリング
推論APIのスケーリングには、GunicornやUvicornの活用、負荷分散の設定が推奨されます。
# Gunicornを使ったAPIスケーリング例
gunicorn -w 4 -k uvicorn.workers.UvicornWorker myapi:app --bind 0.0.0.0:8000
1.3 キャッシュ戦略
Redisキャッシュを活用して、推論結果を保存し、APIの応答速度を改善します。
# Redisを使ったキャッシュの実装例
import redis
cache = redis.Redis(host="localhost", port=6379, db=0)
@app.post("/predict")
async def predict(input_text: str):
cached_response = cache.get(input_text)
if cached_response:
return {"response": cached_response.decode()}
input_ids = tokenizer.encode(input_text, return_tensors="pt")
output = model.generate(input_ids, max_length=50)
response_text = tokenizer.decode(output[0], skip_special_tokens=True)
cache.set(input_text, response_text)
return {"response": response_text}
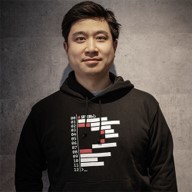
下田 昌平
開発と設計を担当。1994年からプログラミングを始め、今もなお最新技術への探究心を持ち続けています。カテゴリー
検索履歴
チーム
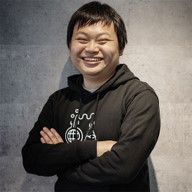
任 弘毅
株式会社レシートローラーにて開発とサポートを担当。POSレジやShopifyアプリ開発の経験を活かし、業務のデジタル化を促進。
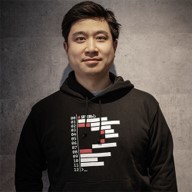
下田 昌平
開発と設計を担当。1994年からプログラミングを始め、今もなお最新技術への探究心を持ち続けています。